Qwiic Ultrasonic Distance Sensor (HC-SR04) Hookup Guide
Software Setup and Programming
Our friends over at Zio have provided an example to get you started with this Ultrasonic Distance Sensor. In order to do so, you'll need to install a few libraries first.
To display the sensor readings on the connected Qwiic OLED, we will use three Adafruit libraries:
Adafruit BusIO Library
You can install this library to automatically in the Arduino IDE's Library Manager by searching for "Adafruit BusIO". Or you can manually download it from the GitHub repository.
Adafruit GFX Library
You can install this library to automatically in the Arduino IDE's Library Manager by searching for "Adafruit GFX". Or you can manually download it from the GitHub repository.
Adafruit SSD1306 Library
You can install this library to automatically in the Arduino IDE's Library Manager by searching for "Adafruit SSD1306 Library". Or you can manually download it from the GitHub repository.
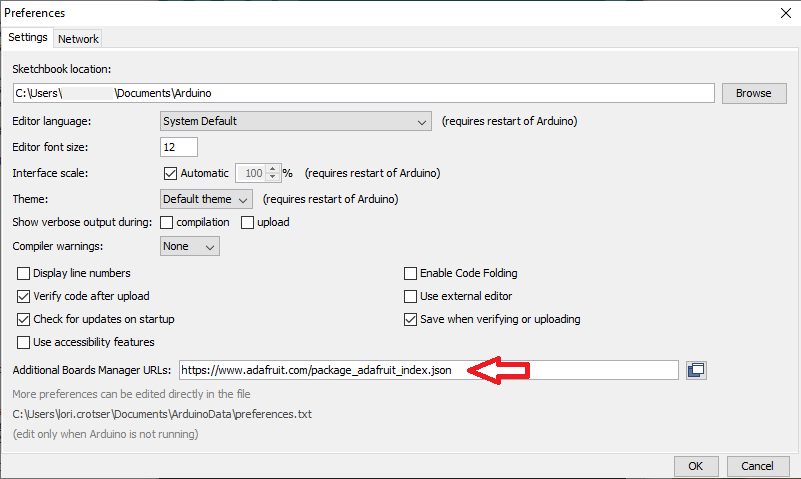
Example 1
This example lives in the GitHub Repo in the Arduino folder. Feel free to download the code, alternatively you can copy the code below into a blank Arduino sketch. Select your board (for this example we'd select "SparkFun RedBoard") and the port your board has enumerated on. Go ahead and upload your code.
language:c
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define SLAVE_BROADCAST_ADDR 0x00 //default address
#define SLAVE_ADDR 0x00 //SLAVE_ADDR 0xA0-0xAF
uint8_t distance_H = 0;
uint8_t distance_L = 0;
uint16_t distance = 0;
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 32 // OLED display height, in pixels
// Declaration for an SSD1306 display connected to I2C (SDA, SCL pins)
#define OLED_RESET 4 // Reset pin # (or -1 if sharing Arduino reset pin)
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
void setup() {
Wire.begin(); // join i2c bus (address optional for master)
Serial.begin(9600); // start serial for output
display.begin(SSD1306_SWITCHCAPVCC, 0x3C); // initialize with the I2C addr 0x3C (for the 128x32)
Serial.println("IIC testing......");
display.clearDisplay();
// Wire.beginTransmission(SLAVE_BROADCAST_ADDR); // transmit to device SLAVE_BROADCAST_ADDR
// Wire.write(SLAVE_ADDR); // Change the SLAVE_ADDR
// Wire.endTransmission(); // stop transmitting
}
void loop() {
Wire.beginTransmission(SLAVE_ADDR); // transmit to device #8
Wire.write(1); // measure command: 0x01
Wire.endTransmission(); // stop transmitting
Wire.requestFrom(SLAVE_ADDR, 2); // request 6 bytes from slave device #8
while (Wire.available()) { // slave may send less than requested
distance_H = Wire.read(); // receive a byte as character
distance_L = Wire.read();
distance = (uint16_t)distance_H << 8;
distance = distance | distance_L;
Serial.print(distance); // print the character
Serial.println("mm");
display.setTextSize(2);
display.setTextColor(WHITE);
display.setCursor(10, 0);
display.clearDisplay();
display.print(distance);
display.print("mm");
display.display();
delay(1);
delay(100);
}
}
Try moving an object (like your hand or a dinosaur) closer to the sensor - notice the output of the OLED shows you how close the object is! Grr. Rawr!